Java objects reside in a memory area called the heap. It is possible to know the memory specifications of heap in a Java program. In this blog, I’ll demonstrate how to find heap size in Java using the java.lang.Runtime
class.
java.lang.Runtime
class has the following 3 methods which can give information about the heap memory size:
totalMemory()
– Returns the total amount of memory in the Java virtual machine.maxMemory()
– Returns the maximum amount of memory that the Java virtual machine will attempt to use.freeMemory()
– Returns the amount of free memory in the Java Virtual Machine.
HeapSizeDemo.java
public class HeapSizeDemo { public static void main(String[] args) { java.io.PrintStream out = System.out; // Get an instance of the Runtime class Runtime runtime = Runtime.getRuntime(); // To convert from Bytes to MegaBytes: // 1 MB = 1024 KB and 1 KB = 1024 Bytes. // Therefore, 1 MB = 1024 * 1024 Bytes. long MegaBytes = 1024 * 1024; // Memory which is currently available for use by heap long totalMemory = runtime.totalMemory() / MegaBytes; out.println("Heap size available for use -> " + totalMemory + " MB"); // Maximum memory which can be used if required. // The heap cannot grow beyond this size long maxMemory = runtime.maxMemory() / MegaBytes; out.println("Maximum memory Heap can use -> " + maxMemory + " MB"); // Free memory still available long freeMemory = runtime.freeMemory() / MegaBytes; out.println("Free memory in heap -> " + freeMemory + " MB"); // Memory currently used by heap long memoryInUse = totalMemory - freeMemory; out.println("Memory already used by heap -> " + memoryInUse + " MB"); } }
A sample output of the above program is shown below
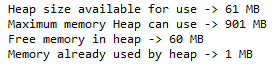
Note
The methods in the
The methods in the
java.lang.Runtime
class return the size of memory in bytes.
How to find Heap size in Java