java.lang.OutOfMemoryError: Java heap space is an error (not an exception) which is thrown by the Java Virtual Machine (JVM) to indicate that it has run out of heap space. You are trying to create new object(s) but the amount of memory required is simply not available on the heap.
Now the question arises, why didn’t the JVM call the Garbage Collector (GC) and free up memory on the heap? The answer is, the JVM has already called the GC to free up some memory on heap but this has still not freed any memory on the heap. You’ve written your program in such a way that the GC cannot find any null references a.k.a unused objects. All your objects are still tied to valid references. Such objects cannot be marked for deletion by the garbage collector. The GC reports its inability to find any free memory to the JVM and the JVM reports this to the programmer/user by throwing the java.lang.OutOfMemoryError Java heap space
Let’s write a program to simulate this kind of memory leak in Java. Create a Java program in Eclipse with the following structure
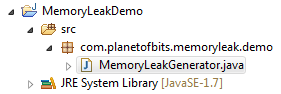
The class MemoryLeakGenerator.java
in the demo program creates integer
arrays of varied lengths in an infinite loop. Since arrays in Java require contiguous memory on heap, there will a point when the JVM will not find any free contiguous memory area of the desired length. This will ultimately result in a memory leak and the JVM will throw java.lang.OutOfMemoryError: Java heap space
package com.planetofbits.memoryleak.demo; public class MemoryLeakGenerator { public void createArrays() { int arraySize = 20; // Create arrays in an infinite loop while (true) { System.out.println("Available memory (in bytes): " + Runtime.getRuntime().freeMemory()); int[] fillMemory = new int[arraySize]; arraySize = arraySize * 5; } } public static void main(String[] args) throws Exception { MemoryLeakGenerator leakGenerator = new MemoryLeakGenerator(); leakGenerator.createArrays(); } }
Following is the output generated on the execution of the above program
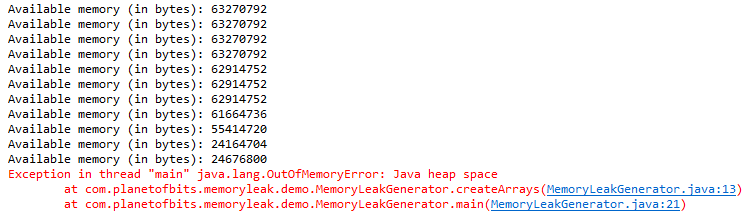