Memory management is the process of recognizing when allocated objects are no longer needed, deallocating (freeing) the memory used by such objects, and making it available for subsequent allocations.
In Java, memory management is the responsibility of program called the garbage collector (commonly called as GC). The garbage collector in Java, automatically runs at regular intervals to perform memory management activities. Let’s see how to call garbage collector in Java program. The objective of this demo is to get familiar with the process of garbage collection.
Please note that a program should not call the garbage collector explicitly. Even if the garbage collector is called in a program, it is not certain that it will run at that very moment. Calling the garbage collector is a request (not a command) to the JVM to invoke the process. It is the JVM which decides when to run the garbage collector. Running the garbage collector explicitly when a program is in execution is a resource intensive job.
A Java project is created in Eclipse with the following structure
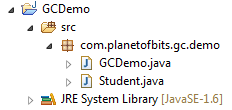
The code for the GCDemo.java
and Student.java
classes are as follows
package com.planetofbits.gc.demo; import java.io.IOException; import java.util.ArrayList; public class GCDemo { public static void main(String[] args) throws IOException { java.io.PrintStream out = System.out; // Get the Runtime instance of the JVM Runtime rt = Runtime.getRuntime(); out.println("--- Initial state of memory ---"); // Returns the maximum amount of memory that the Java virtual // machine will attempt to use. out.println("Maximum memory (in bytes): " + rt.maxMemory()); // Returns the total amount of memory in the Java virtual machine. out.println("Total memory (in bytes): " + rt.totalMemory()); // Returns the amount of free memory in the Java Virtual out.println("Free memory (in bytes): " + rt.freeMemory()); ArrayListstudentList = new ArrayList (); for (int i = 1; i <= 1000; i++) { Student student = new Student(); student.setRollNo(i); student.setName("S" + i); studentList.add(student); } out.println("\n--- After creating the ArrayList ---"); long freeMemoryBeforeGC = rt.freeMemory(); out.println("Free memory (in bytes): " + freeMemoryBeforeGC); // Removes all of the elements from this list. // The list will be empty after this call returns. studentList.clear(); // Null reference the ArrayList handle studentList = null; // Runs the finalization methods of any objects pending finalization. rt.runFinalization(); out.println("\n--- Garbage Collector invoked ---"); // Call the garbage collector rt.gc(); out.println("\n--- After garbage collection ---"); long freeMemoryAfterGC = rt.freeMemory(); out.println("Free memory (in bytes): " + freeMemoryAfterGC); out.println("Memory freed by GC (in bytes): " + (freeMemoryAfterGC - freeMemoryBeforeGC)); } }
System.gc();
is another way to invoke the garbage collector. However, if we look inside the java.lang.System.gc()
method, it internally calls the Runtime.getRuntime().gc()
It is more of a convenience to call
System.gc()
with no technical difference. In this case, gc()
is a class method of java.lang.System
whereas in java.lang.Runtime
, it is an instance method.
package com.planetofbits.gc.demo; public class Student { private int rollNo; private String name; public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
To view what the Garbage Collector is doing when it is invoked, pass -verbose:gc to JVM as shown below
Step 1
Open Eclipse and in the toolbar menu, go to Run -> Run Configurations...
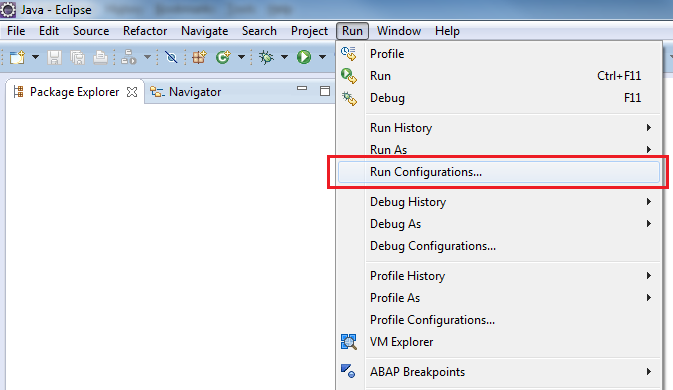
Step 2
In the left pane of Run Configurations window, navigate to the Java Application node and select the Java application for which you need to increase the heap size. Then in the right pane, click on the Arguments tab.
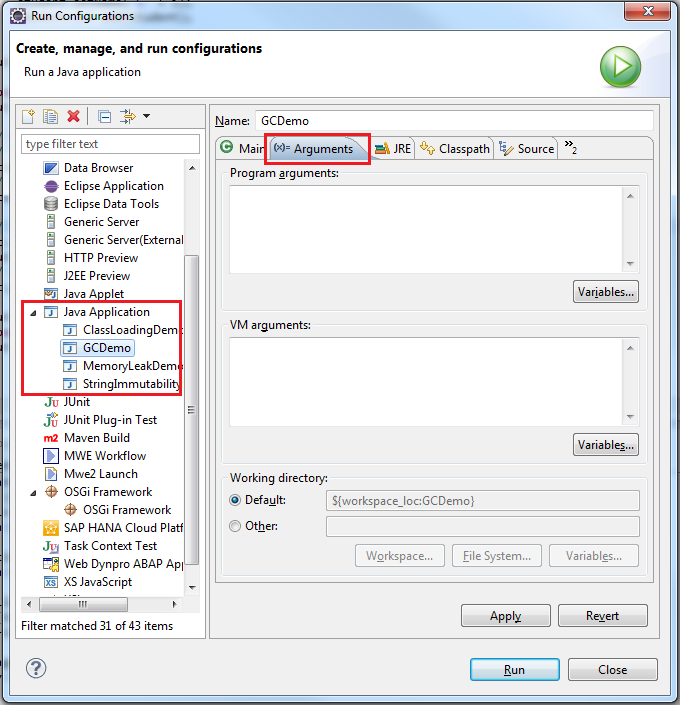
In the VM Arguments section, type the following argument:
-verbose:gc
Click on Apply button to save the changes.
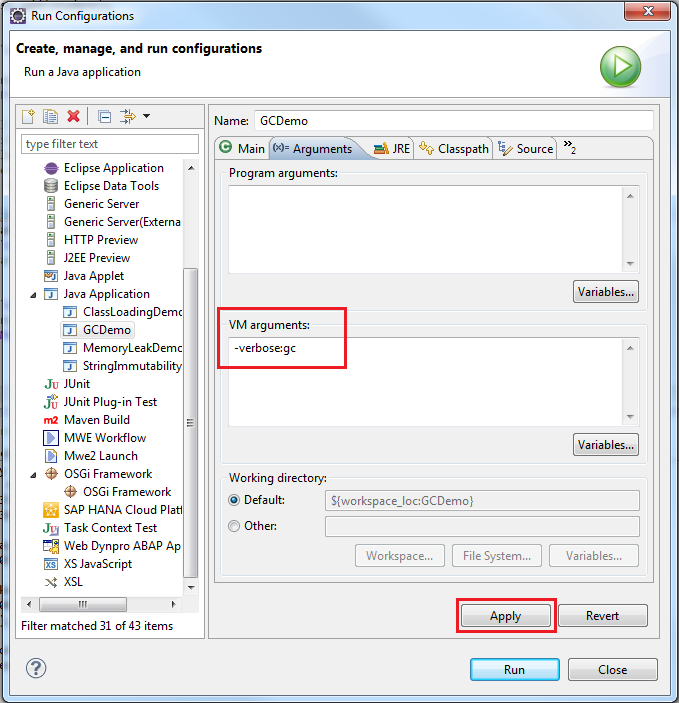
Running the GCDemo.java
class gives the following output on my machine.
Please not that the output of this program depends on the platform environment in which this program is executed. If you execute this code on your machine, it will not match with the output shown below.
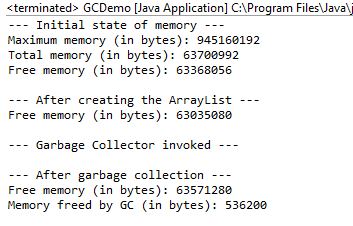
In the above output, the free memory after garbage collection is higher than the initial memory. This indicates that some memory has been reclaimed by the garbage collection program. However, as already said, please be aware that there might be a completely different output on your machine.